728x90
반응형
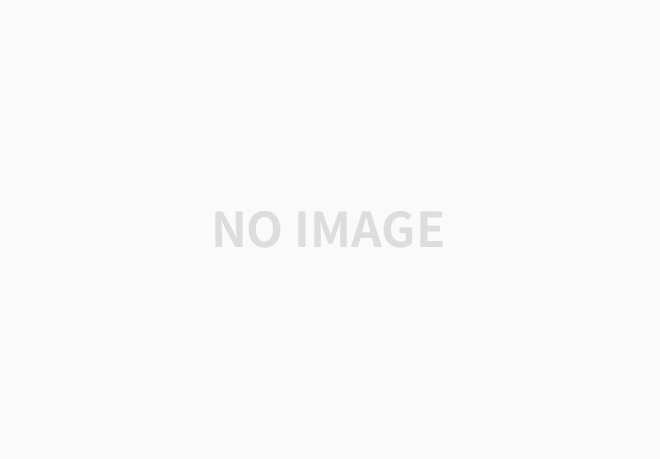
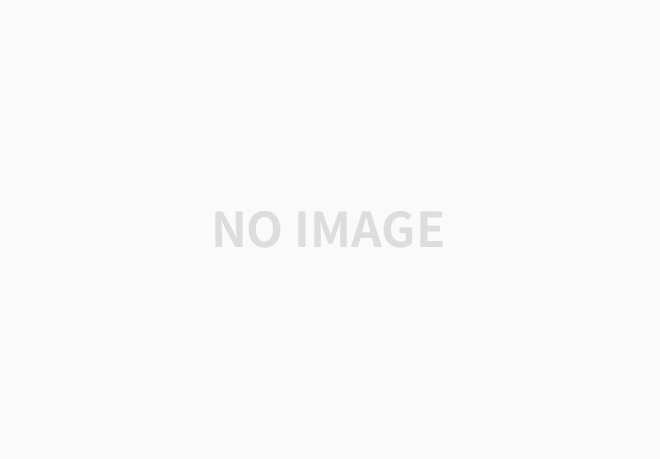
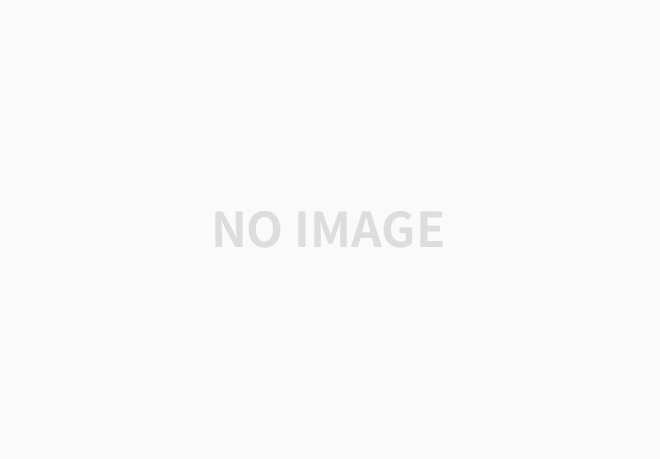
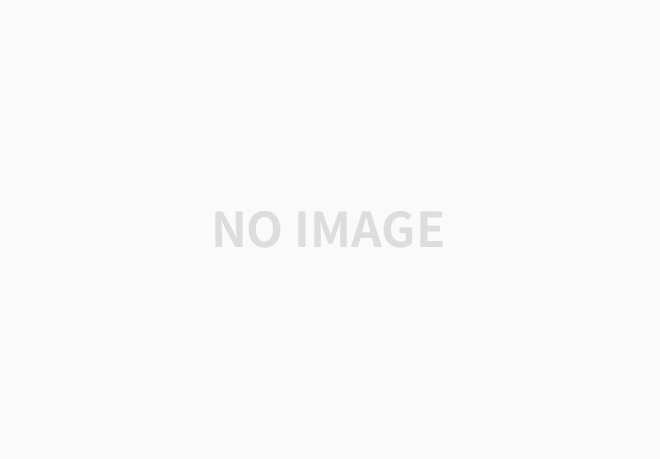
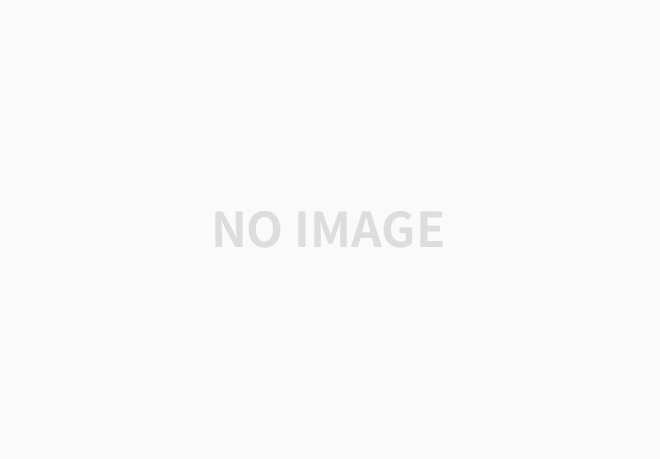
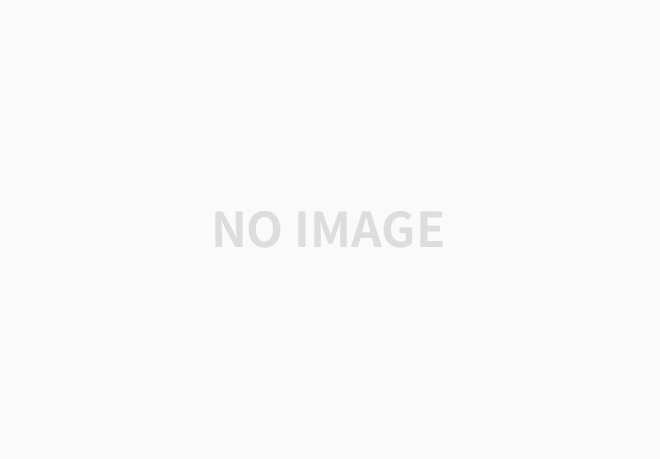
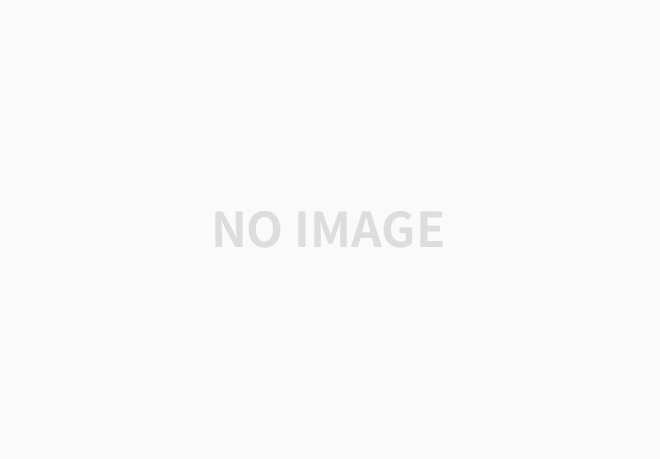
**소스**
MainActivity.java
package com.threedpit.myservice;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editText);
Button btn = findViewById(R.id.button);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = editText.getText().toString();
Intent intent = new Intent(getApplicationContext(),MyService.class);
intent.putExtra("command","show");
intent.putExtra("name",name);
//지금까지 우리가 startActivity를 했었는데 그것은 화면을 띄우는경우
// 지금은 서비스를 하는것 이기 때문에 StartService();로 한다.
startService(intent);
}
});
}
}
**activity_main.xml**
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPersonName" />
<Button
android:id="@+id/button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="서비스 시작하기" />
</LinearLayout>
**MyService.java**
package com.threedpit.myservice;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.util.Log;
public class MyService extends Service {
//서비스는 비정상동작시 종료되면 다시 실행됨
private static final String TAG = "MyService";// 여러번 입력할것 상수롤 만들어준것
public MyService() {
}
@Override
public void onCreate() {
super.onCreate();
Log.d(TAG,"onCreate 호출됨");
}
@Override
public void onDestroy() {
super.onDestroy();
Log.d(TAG,"onDestory 호출됨");
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Log.d(TAG,"onStartCommand 호출됨");
if(intent != null) {
processCommand(intent); //함수를 만들어서 그 인텐트를 처리 하겠다 하는것
}else{
return Service.START_STICKY; //이 명령어가 자동으로 시작해라 하는것
}
return super.onStartCommand(intent, flags, startId);
}
@Override
public IBinder onBind(Intent intent) {
// TODO: Return the communication channel to the service.
throw new UnsupportedOperationException("Not yet implemented");
}
public void processCommand(Intent intent){
String command =intent.getStringExtra("command");
String name = intent.getStringExtra("name");
Log.d(TAG,"command : "+command+"name : " +name);
}
}
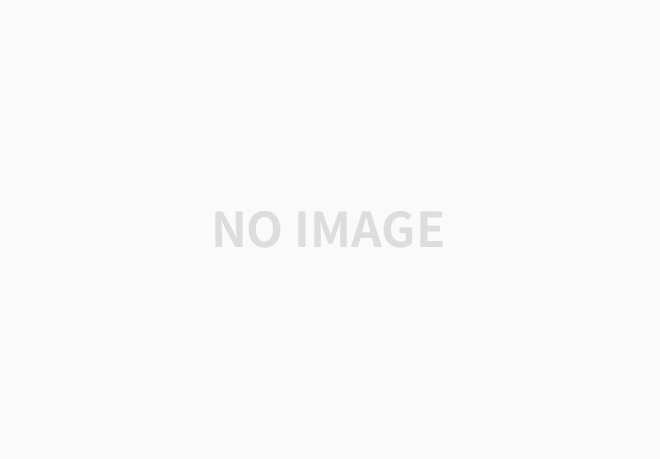
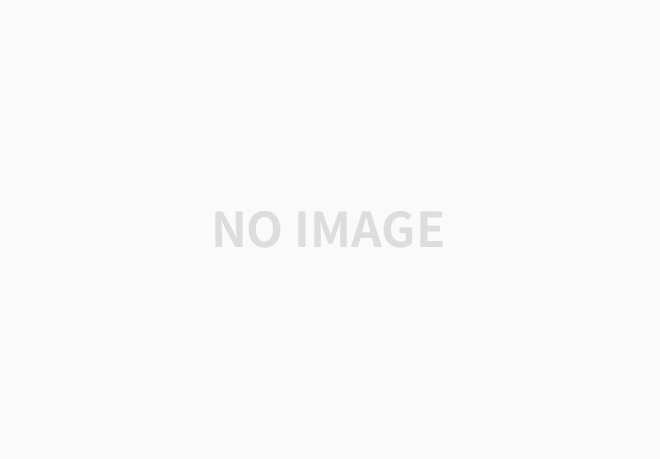
**MyService.java**
package com.threedpit.myservice;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.util.Log;
public class MyService extends Service {
//서비스는 비정상동작시 종료되면 다시 실행됨
private static final String TAG = "MyService";// 여러번 입력할것 상수롤 만들어준것
public MyService() {
}
@Override
public void onCreate() {
super.onCreate();
Log.d(TAG,"onCreate 호출됨");
}
@Override
public void onDestroy() {
super.onDestroy();
Log.d(TAG,"onDestory 호출됨");
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Log.d(TAG,"onStartCommand 호출됨");
if(intent != null) {
processCommand(intent); //함수를 만들어서 그 인텐트를 처리 하겠다 하는것
}else{
return Service.START_STICKY; //이 명령어가 자동으로 시작해라 하는것
}
return super.onStartCommand(intent, flags, startId);
}
@Override
public IBinder onBind(Intent intent) {
// TODO: Return the communication channel to the service.
throw new UnsupportedOperationException("Not yet implemented");
}
public void processCommand(Intent intent){
String command =intent.getStringExtra("command");
String name = intent.getStringExtra("name");
Log.d(TAG,"command : "+command+"name : " +name);
// -------------------------------------추가 부분--------------------------------
try {
Thread.sleep(5000);//5초동안 쉬는것
}catch (Exception e){
e.printStackTrace();
}
Intent showIntent = new Intent(getApplicationContext(),MainActivity.class);
//아래의 flags가 없다면 이미 현재 메인 액티비티 화면에서 저게 실행이되는것 이라
//현재의 같은 화면이 또 뜨게 된다.
//SINGLE_TOP은 이미 메인엑티비티가 만들어져 있으면 그것을 그대로 보여주라고 하는것이고
//CLEAR_TOP은 여러개 쌓여있으면 위에 것을 없애라 하는 것
showIntent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK|
Intent.FLAG_ACTIVITY_SINGLE_TOP|
Intent.FLAG_ACTIVITY_CLEAR_TOP );
//NEW_TASK라고 하는것은 화면이 하나의 앱에서 계속 연속적으로 보이는것 처럼 만들어 줄때
//TASK라는게 무조건 만들어 진다. 화면이 없는 상태에서 화면을 보여주고 싶을때도 만들어 줘야함
showIntent.putExtra("command","show");//우리가 전달 받은것을 다시 보내기
showIntent.putExtra("name",name+"from service");
//메인 액티비티 쪽에서 위의 인텐트를 받아서 처리할 수 있어야하는데
// 그 인텐트를 받아서 처리하는 방법은 메인액티비티.java에 서
startActivity(showIntent);
// -------------------------------------추가 부분--------------------------------
}
}
**MainActivity.java**
package com.threedpit.myservice;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
EditText editText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editText = findViewById(R.id.editText);
Button btn = findViewById(R.id.button);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = editText.getText().toString();
Intent intent = new Intent(getApplicationContext(),MyService.class);
intent.putExtra("command","show");
intent.putExtra("name",name);
//지금까지 우리가 startActivity를 했었는데 그것은 화면을 띄우는경우
// 지금은 서비스를 하는것 이기 때문에 StartService();로 한다.
startService(intent);
}
});
// ---------------------------- 추가 부분----------------------------------
Intent intent = getIntent();
processIntent(intent);
}
@Override
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
processIntent(intent);
}
public void processIntent(Intent intent){
// 문제는 이미 메인액티비티가 메인 메모리에 만들어져 있는 상태인경우
//onCreate가 실행이 되지 않는다.그래서 그것 대신에 위에onNewIntent이 호출된다.
// 저렇게 onNEwIntent를 선언하고
if(intent !=null){
String command = intent.getStringExtra("command");
String name = intent.getStringExtra("name");
Toast.makeText(this,"command : "+command+"name :"+name,Toast.LENGTH_LONG).show();
}
}
// ---------------------------- 추가 부분----------------------------------
}
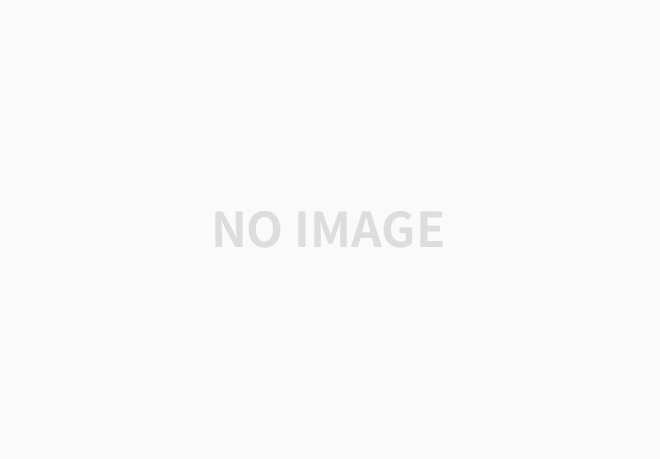
728x90
반응형
'안드로이드(Android)' 카테고리의 다른 글
안드로이드 이론 빡공 18 (0) | 2020.07.01 |
---|---|
안드로이드 이론 빡공 17 (0) | 2020.06.29 |
안드로이드 이론 빡공 15 (0) | 2020.06.23 |
안드로이드 이론 빡공 14 (0) | 2020.06.22 |
안드로이드 이론 빡공 13 (0) | 2020.06.19 |
댓글